CompoundView란?
→ 비슷한 뷰를 반복해서 사용해야할 때 유용
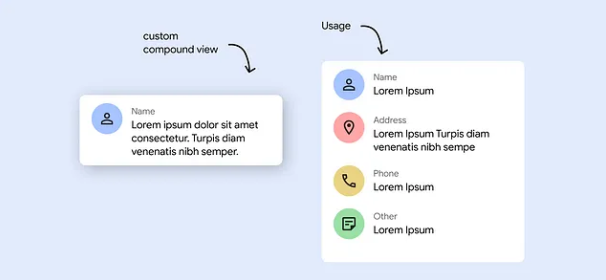
Compound View 만들기
- 뷰만들기
<?xml version="1.0" encoding="utf-8"?>
<merge xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<ImageView
android:id="@+id/icon"
android:layout_width="32dp"
android:layout_height="32dp"
android:background="@drawable/circular_bkg"
android:padding="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:srcCompat="@drawable/ic_outline_people_24" />
<TextView
android:id="@+id/text_title"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="12dp"
android:textColor="?android:textColorSecondary"
android:textSize="12sp"
app:layout_constraintBottom_toTopOf="@+id/text_value"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toEndOf="@+id/icon"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_chainStyle="packed"
tools:text="Lorem Ipsum" />
<TextView
android:id="@+id/text_value"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="12dp"
android:layout_marginTop="0dp"
android:textColor="?android:textColorPrimary"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toEndOf="@+id/icon"
app:layout_constraintTop_toBottomOf="@+id/text_title"
tools:text="Lorem Ipsum" />
</merge>
- 기존 viewgroup 확장하는 사용자 지정 view class만들기
public class CompoundListItem extends ConstraintLayout {
public CompoundListItem(Context context) {
this(context, null);
}
public CompoundListItem(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
}
}
- 커스텀 속성 정의
<resources>
<declare-styleable name="CompoundListItem">
<attr name="titleText" format="string" />
<attr name="valueText" format="string" />
<attr name="icon" format="reference" />
<attr name="iconTint" format="color" />
<attr name="iconBackground" format="reference" />
</declare-styleable>
</resources>
- 사용자 정의 속성 적용
.....
private TextView txtTitle, txtValue;
private ImageView image;
...
public CompoundListItem(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
inflater.inflate(R.layout.compound_list_item, this, true);
TypedArray typedArray = context.obtainStyledAttributes(
attrs,
R.styleable.CompoundListItem,
0,
0
);
String titleText = a.getString(R.styleable.CompoundListItem_titleText);
String valueText = a.getString(R.styleable.CompoundListItem_valueText);
Drawable icon = a.getDrawable(R.styleable.CompoundListItem_icon);
int iconTint = a.getColor(R.styleable.CompoundListItem_iconTint,getResources().getColor(R.color.white));
Drawable iconBackground = a.getDrawable(R.styleable.CompoundListItem_iconBackground);
typedArray.recycle();
image = findViewById(R.id.icon);
txtTitle = findViewById(R.id.text_title);
txtValue = findViewById(R.id.text_value);
setTxtTitle(titleText);
setTxtValue(valueText);
setImage(icon);
setImageTint(iconTint);
setImageBackground(iconBackground);
}
- 속성, 이벤트 추가
public void setTxtTitle(String txt) {
txtTitle.setText(txt);
}
public void setTxtValue(String txt) {
txtValue.setText(txt);
}
public void setImage(Drawable icon) {
image.setImageDrawable(icon);
}
public void setImageBackground(Drawable background) {
image.setBackground(background);
}
public void setImageTint(int color) {
image.setImageTintList(ColorStateList.valueOf(color));
}
- 사용
<com.yourpackagename.widgets.CompoundListItem
android:id="@+id/tv_dummy"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginVertical="8dp"
app:icon="@drawable/ic_outline_article_24"
app:iconBackground="@drawable/circular_bkg"
app:titleText="Test"
app:valueText="sample text with note" />
Uploaded by N2T